How GIT works in project ?
1. Set up a project locally, initialise git (make the project folder a git repository).
2. add all the changed files of project in GIT.
3. COMMIT changes in GIT.
4. then push local repository.
5. Make a PR/MR towards your master/develop branch in remote github.com or gitlab.com or bitbucket.com and then after review merge it.
Some exceptions like merge conflict may occur and different situations like rolling back some changes etc which will be dealt also but lets study above process in detail with corresponding commands.
GIT has 3 conceptual tables and all commands push changes from one table to other, while clearing the earlier table. The 3 tables are: Local Git Table, Staging table and remote table.,
Now we will integrate git commands and concepts along with git tables concept. It is extremely important to master GIT.
1. Initialise GIT:
It means make a local project in your local system a git repository. It can be done only once. The purpose of doing this is so that many people can work on the same project by sharing the codebase and merging changes together while working parallelly. Now we can anytime make this project opt out of being git repository also, deinitialise a repository.
// initialise a git repository git init // deinitialise a git repository rm -rf .git // .git files stores metadata so remove it
2. Add all the changed files in GIT:
After making the project a git repository in earlier step, now you might want to write a feature or do some code changes in your project. Once you finish your work, then add all of those changed files in GIT by below command. And this command will move all the changes into Local GIT table too.
If you run the command git status before firing below command, then in terminal all the changed files will look in dark red color. But if you run the same command after firing below command, then in terminal all the changed files will look in dark green color.
git add .
3. Commit changes in GIT:
Commit means grouping changes in above files under a user given description describing the purpose for changing the files. Whenever we push or pull our changes from remote repository, we infact do not push/pull the entire project but only the commits or group of changes in files or folders.
Next, commit all of those changed files in GIT by below command.
And this command will move all the added changes from local git table into Staging table and make the previous table empty.
If you run the command git status before firing below command, then in terminal all the changed files will look in dark green color. But if you run the same command after firing below command, then in terminal nothing will appear in terminal except a message that working tree clean.
git commit -m "Created a Navbar" // Inside double quote is the commit message that user can write
4. Make a remote repository:
Remote or remote repository means repository on https://github.com/ or https://bitbucket.org/ or other servers. Go on any URL, here we go to github.com and then signup and login with username and password. Then click on Create a New repository and then give a proper name of repository. Once you click on create button then some commands will appear but we are interested only in adding remote address command and then pushing command.
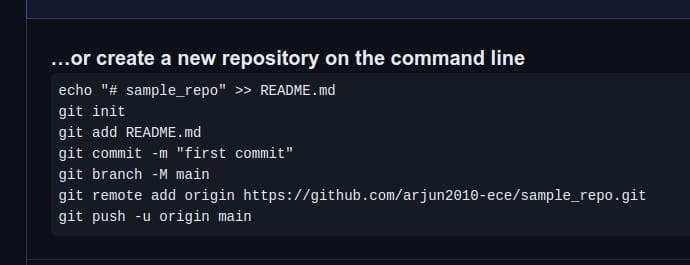
5. Push local changes to server: In the above image, we find a lot of commands but we will use only 3 commands, but first command is not mandatory. Second and third is mandatory. And third command is what really pushes the branch on remote.
Also this command pushes change into remote table too, so even if our system crashes or any data is lost locally our changes will not be impacted and we can access it anytime from server.
git branch -M main // change the name of current local branch to main git remote add origin https://github.com/arjun2010-ece/sample_repo.git // add remote address locally git push -u origin main // Push local branch on remote github.com server
Steps done by normal developers :
Now that was for the first time push and was mostly done by a Team Lead or senior developer who pushed our standard branch i.e main branch in this case to remote. Now everyone in the team will first clone the repository into our local system and then make a new branch out of that locally based on the feature your are going to write e.g "creating a navbar" so branch can be named as "feature/creating_navbar", write our changes into that repository. Then add those files into git (as shown in above) and then commit and then push as shown (while pushing branch name will be "feature/creating_navbar"). This push will push local branch into remote.
git clone https://github.com/arjun2010-ece/sample_repo.git // add remote address locally git checkout -b feature/creating_navbar git add . git commit -m "created the navbar" git push -u origin feature/creating_navbar // Push local branch on remote github.com server
5. Make a PR/MR on github.com or bitbucket : PR(Pull request) or MR(Merge request) means same thing and that is merging changes(or commits) from your remote feature branch into master/develop/main branch (where everyone merges their code and pulls the fresh copy to write new features).
Now once we push our local branch on remote as shown above, now we got to github.com and on our project repository and find the oprion of creating a New Pull request or Merge request and then select sourch branch as our feature branch and destination branch as our standard branch i.e main(in this case) but can be develop/master or anything also and then create it.
If you work in a team then at this stage you ask your senior to review it and merge it. If you work alone then you will have to merge it. And this merge will merge the commits or changed files into remote standard branch i.e main here.
Now everytime you have to write a new feature, you are going to follow the steps as mentioned in "Steps done by normal developers" only.