Grid Properties
In Grid system (same as flexbox), we have a grid parent (or container) and grid childs. By applying few properties on grid parent, we control how the child will lay on html page.
Once we apply display: grid on a parent element, then all its child becomes grid child and then we can control its child through some grid properties, defined below
And these properties are applied on its parent itself.
We use some specific and limited properties on its childs, which we study in next page but here we study properties on parents only.
The most common properties always used are: grid-template-columns, grid-template-rows justify-content, align-items property and gap property.
And these justify-content and align-items property are the same as used in flexbox in previous section.
Now grid is a concept of rows and columns. As shown in below, a very basic grid we have drawn with 4 rows and 3 columns (although we can draw with any number of rows/columns) looks like this, with spaces between them (called gaps/gutters) may or may not exists::
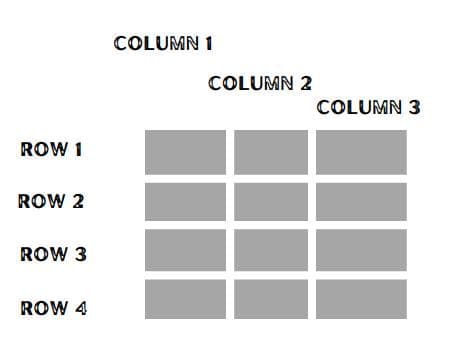
Grid templates columns:
Grid template columns is a property which is used to create number of columns in a grid.
Now since grid is a combination of rows and columns. Now what about rows ??.
Either we set it with other property (will discuss next), and if not set then number of rows will
be automatically created ensuring that number of columns (as created above by the property) is always
respected.
For ex: please observe the html snippet below, here once we apply the grid css in it, we will have
2 grid columns of 400px and 600px. Now regarding number of rows will be 3. Just calculate it in
your mind.
Now you can look at the results of below html css here:
result
// HTML <div class="container" > <div class="item" ></div> <div class="item" ></div> <div class="item" ></div> <div class="item" ></div> <div class="item" ></div> <div class="item" ></div> </div> //css .container{ display: grid; grid-template-columns: 400px 600px; }
Now if you see the css and specifically grid-template-columns value that is 400px
and 600px, that is a static value so once you squeeze the screen or if you see the UI in
smaller screen then you will have horizontal scrollbar which is a bad sign.
To fix that, always use % or fr units for defining column widths.
Note::
If you do not know, how many columns you need to create then in that specific scenario,
you use :
grid-template-columns: repeat(auto-fit, minmax(190px, 1fr));
Here, repeat() is a function which takes 2 values, first value mean how many times to repeat
and second value mean the width of each column or row(depend on where it is used).
For e.g : grid-template-columns: repeat(2, 300px), mean create 2 columns of 300px each.
Also,
grid-template-rows: repeat(2, 400px), mean create 2 rows of 400px each.
auto-fit keyword automatically generates as many columns as it can respecting the
available space. Another keyword used for the same purpose is : auto-fill.
minmax() is a function that takes 2 values i.e minimum and maximum value. In the above code
minmax(190px, 1fr) mean the minimum column width is 190px and maximum is 1fr, meaning the
maximum available space.
So now the above function means that create as many columns as possible(auto-fit) respecting the space
available with a minimum width of 190px and distributing the remaining space evenly
using the 1fr unit.
Popular ways of writing is:
The way to write grid-template-columns is by either repeat() function or by directly putting the width value (either in px/rem or fr or percentage or a mix of both) separated by a space referrring number of columns and width of each column. Some snippets are:
// HTML <div class="container" > <div class="item" >1</div> <div class="item" >2</div> <div class="item" >3</div> <div class="item" >4</div> <div class="item" >5</div> <div class="item" >6</div> </div> //First way .container{ display: grid; grid-template-columns: 400px 600px; // 2 columns of 400 and 600px widths } //Second way .container{ display: grid; grid-template-columns: repeat(2, 1fr); // 2 columns of equal widths } //Third way .container{ display: grid; grid-template-columns: repeat(2, minmax(200px, 1fr)); // 2 columns of minimum widths 200px but max width can be decided by grid itself } //Fourth way .container{ display: grid; grid-template-columns: repeat(2, 300px); // 2 columns of fixed widths 300px } //Fifth way .container{ display: grid; grid-template-columns: repeat(2, 30%); // 2 columns of 30% widths } //Sixth way .container{ display: grid; grid-template-columns: 20% 30% 50%; // 3 columns of 20% , 30% and 50% widths } //Seventh way .container{ display: grid; grid-template-columns: 1fr 2fr 4fr; // 3 columns of 1fr 2fr and 4fr widths }
I am going to explain one very important concept of fr units.
grid-template-columns: 1fr 2fr 4fr
.
Here, how will you understand the space division or width of each columns in fr units.
Adding 1fr + 2fr + 4fr = 7fr units equalling all the available space i.e 100% so,
1fr column width = 100%/7 = 14.287% of available space is the width and so 2fr = 28.574% width and
4fr = 57.148% width of all available space. Hope you understand the fr units well.
Grid templates rows:
Grid template rows is a property which is used to create number of rows in a grid.
but it is not used alone. It is used along with grid-template-columns.
Now we need to be extremely careful while we use grid-template-rows as it will only
be respected when we calculate and put exact value of grid-template-columns
For ex: please observe the html snippet below, here we have 6 grid childs, if we define something
2 rows by grid-template-rows: repeat(2, 300px) of 300px each, then we need to define number
of columns also by mentally calculating what number of columns will respect 2 rows so,
it will be 3 columns.
Now you can look at the results of below html css here:
result
// HTML <div class="container" > <div class="item" >1</div> <div class="item" >2</div> <div class="item" >3</div> <div class="item" >4</div> <div class="item" >5</div> <div class="item" >6</div> </div> //css .container{ display: grid; grid-template-rows: repeat(2, 300px); grid-template-columns: 200px 220px 230px; }
If you do not know, how many rows you need to create then in that specific scenario, well that scenario will never come as you might not know how many columns you need but you will always know how many rows you need or it will be automatically created for you.
Always use % or fr units for responsivity and also can use repeat() function and minmax() function too for rows.
Grid gaps:
Please observe that grid-row-gap and grid-column-gap are the old ways of providing gaps so new ways are row-gap and column-gap.
And we can give both row-gap and column-gap property with a standard property i.e gap. Old property name is grid-gap. An example can be seen here : Grid gaps
// First way .child1{ gap: 20px; } // Second way .child1{ gap: 20px 10px; // row gap is 20px and column gap is 10px. } // Third way, putting gaps separately .child1{ row-gap: 20px; column-gap: 10px; }
Note::
For learning grids, you need to understand only 2 properties well, i.e grid-template-columns and grid-template-rows only. Rest i.e gap and justify-content and align-items property that is definately used here work the same way as it works in flexbox as defined in previous page, so i will not explain here that.
Also these properties are not needed as it is rarely used and once you are the master of grids then you can study all of these from official docs
:
- grid-template-areas
- justify-items
- grid-template
- align-content
- place-content
- grid-auto-columns
- grid-auto-rows
- grid-auto-flow